Hey, thanks for the response.
I've been going down the path of creating a box collider pool and like you said the best way to position the tiles is through the tile positioning.
Here's what i'm toying with right now:
(only trying to draw colliders on edges as shown)
it's 100 x 100 tiles
with 863 box colliders on just one game object.
builds in roughly a second, not optimized.
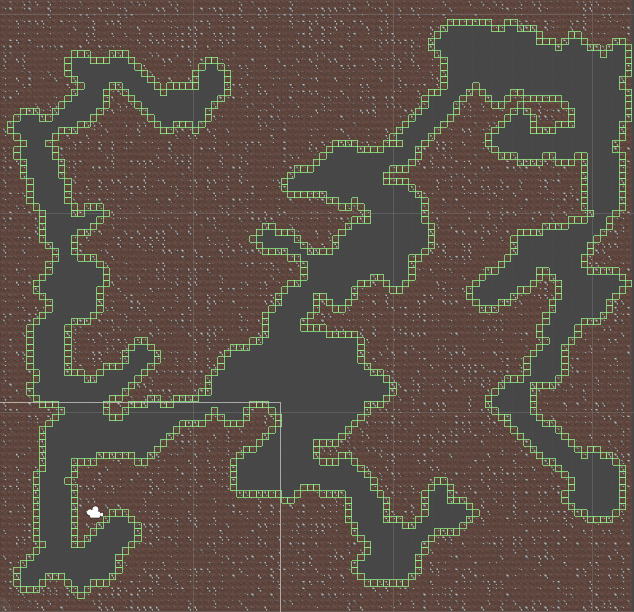
I tried scaling up to 448 x 128 tiles which is 57K tiles with 4700 colliders and the build time is pretty bad, 20 seconds or more. This may be due to naive coding approaches, but I can't help but think that 4700 colliders on one object is probably bad for performance.
I'm still wondering how to get at the chunk object, not the individual tiles, to store the colliders for that chunk so that I can possibly optimize by turning on and off colliders very far from the camera.
tileMap.Layers[layerId].GetChunk(x, y); This returns a type of SpriteChunk. How do I access the GameObject with this? is it actually a GO that I can just use a simple cast or what?
Just to be absolutely clear, I'm saying I want to Isolate the colliders that fall within the chunk and have only those colliders stored on each chunk with the mesh data. pic has the first chunk selected and the colliders.
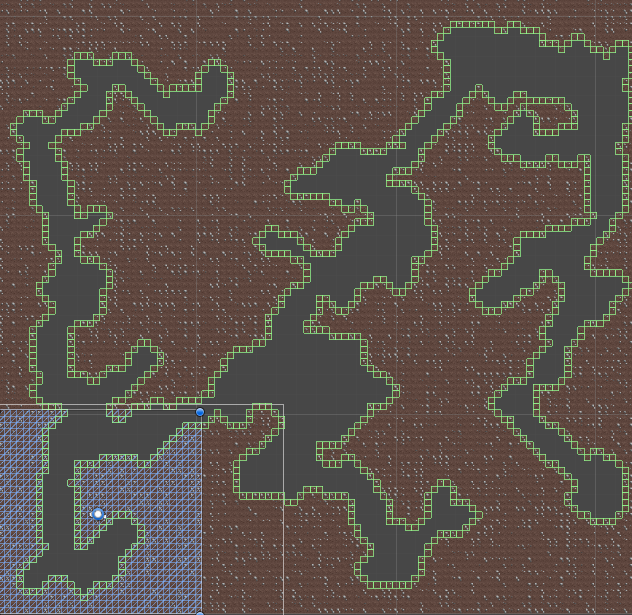